-
Notifications
You must be signed in to change notification settings - Fork 91
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
This creates a basic UI version of the DataGemma notebooks ([rag](https://colab.google.com/github/datacommonsorg/llm-tools/blob/master/notebooks/datagemma_rag.ipynb), [rig](https://colab.google.com/github/datacommonsorg/llm-tools/blob/master/notebooks/datagemma_rig.ipynb)), so that it's easier to play with the two approaches. This is only for internal use and will only be enabled in the local environment and autopush. 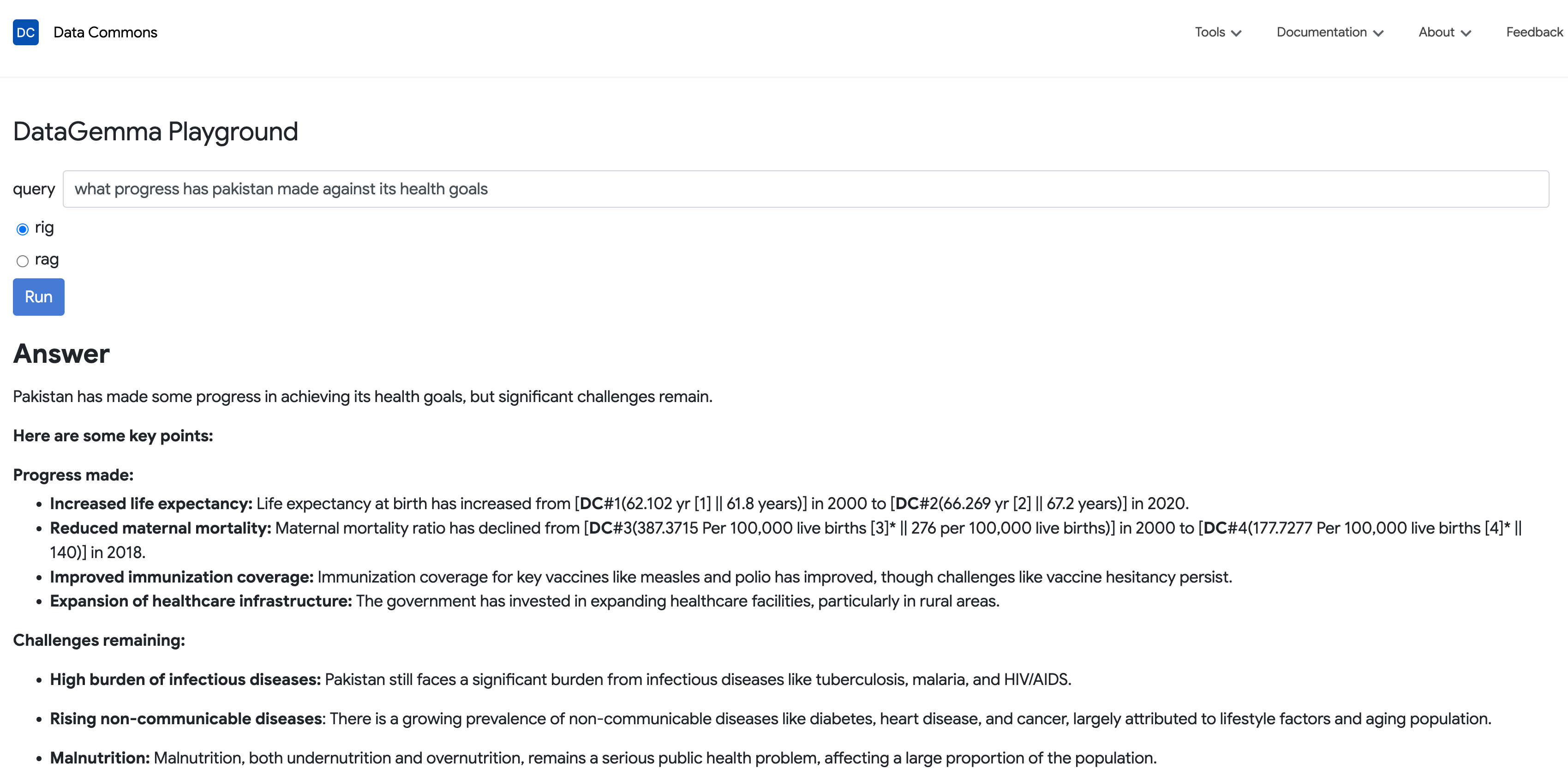 
- Loading branch information
1 parent
bba1b91
commit 6b6b657
Showing
12 changed files
with
540 additions
and
1 deletion.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -25,3 +25,4 @@ class Config(_base.Config): | |
HIDE_DEBUG = False | ||
USE_MEMCACHE = False | ||
ENABLE_BQ = True | ||
ENABLE_DATAGEMMA = True |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,82 @@ | ||
# Copyright 2025 Google LLC | ||
# | ||
# Licensed under the Apache License, Version 2.0 (the "License"); | ||
# you may not use this file except in compliance with the License. | ||
# You may obtain a copy of the License at | ||
# | ||
# http://www.apache.org/licenses/LICENSE-2.0 | ||
# | ||
# Unless required by applicable law or agreed to in writing, software | ||
# distributed under the License is distributed on an "AS IS" BASIS, | ||
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | ||
# See the License for the specific language governing permissions and | ||
# limitations under the License. | ||
"""Endpoints for DataGemma page""" | ||
|
||
import json | ||
|
||
from data_gemma import DataCommons | ||
from data_gemma import GoogleAIStudio | ||
from data_gemma import RAGFlow | ||
from data_gemma import RIGFlow | ||
from data_gemma import VertexAI | ||
import flask | ||
from flask import current_app | ||
from flask import request | ||
from flask import Response | ||
|
||
# Define blueprint | ||
bp = flask.Blueprint('dev_datagemma_api', | ||
__name__, | ||
url_prefix='/api/dev/datagemma') | ||
|
||
_RIG_MODE = 'rig' | ||
_RAG_MODE = 'rag' | ||
|
||
# TODO: consider moving these specifications to a config somewhere | ||
_VERTEX_AI_RIG = VertexAI(project_id='datcom-website-dev', | ||
location='us-central1', | ||
prediction_endpoint_id='4999251772590522368') | ||
_VERTEX_AI_RAG = VertexAI(project_id='datcom-website-dev', | ||
location='us-central1', | ||
prediction_endpoint_id='3459865124959944704') | ||
|
||
|
||
def _get_datagemma_result(query, mode): | ||
"""Gets the results of running a datagemma flow on a query | ||
Args: | ||
query: Query to run datagemma flow on | ||
mode: mode to run the datagemma flow in | ||
Returns: | ||
Results of running the datagemma flow. This is a FlowResponse as defined | ||
here: https://github.com/datacommonsorg/llm-tools/blob/main/data_gemma/base.py#L116 | ||
""" | ||
dc_nl_service = DataCommons(api_key=current_app.config['DC_NL_API_KEY']) | ||
result = None | ||
if mode == _RIG_MODE: | ||
result = RIGFlow(llm=_VERTEX_AI_RIG, | ||
data_fetcher=dc_nl_service).query(query=query) | ||
elif mode == _RAG_MODE: | ||
gemini_model = GoogleAIStudio( | ||
model='gemini-1.5-pro', api_keys=[current_app.config['GEMINI_API_KEY']]) | ||
result = RAGFlow(llm_question=_VERTEX_AI_RAG, | ||
llm_answer=gemini_model, | ||
data_fetcher=dc_nl_service).query(query=query) | ||
return result | ||
|
||
|
||
@bp.route('/query') | ||
def datagemma_query(): | ||
query = request.args.get('query') | ||
mode = request.args.get('mode') | ||
if not query: | ||
return 'error: must provide a query field', 400 | ||
if not mode or mode not in [_RIG_MODE, _RAG_MODE]: | ||
return f'error: must provide a mode field with values {_RIG_MODE} or {_RAG_MODE}', 400 | ||
dg_result = _get_datagemma_result(query, mode) | ||
result = {'answer': '', 'debug': ''} | ||
if dg_result: | ||
result = {'answer': dg_result.answer(), 'debug': dg_result.debug()} | ||
return Response(json.dumps(result), 200, mimetype='application/json') |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,24 @@ | ||
# Copyright 2025 Google LLC | ||
# | ||
# Licensed under the Apache License, Version 2.0 (the "License"); | ||
# you may not use this file except in compliance with the License. | ||
# You may obtain a copy of the License at | ||
# | ||
# http://www.apache.org/licenses/LICENSE-2.0 | ||
# | ||
# Unless required by applicable law or agreed to in writing, software | ||
# distributed under the License is distributed on an "AS IS" BASIS, | ||
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | ||
# See the License for the specific language governing permissions and | ||
# limitations under the License. | ||
"""DataGemma page routes""" | ||
|
||
import flask | ||
|
||
# Define blueprint | ||
bp = flask.Blueprint("dev-datagemma", __name__, url_prefix='/dev/datagemma') | ||
|
||
|
||
@bp.route('/') | ||
def dev_datagemma(): | ||
return flask.render_template('dev/datagemma.html') |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
{# | ||
Copyright 2025 Google LLC | ||
|
||
Licensed under the Apache License, Version 2.0 (the "License"); | ||
you may not use this file except in compliance with the License. | ||
You may obtain a copy of the License at | ||
|
||
http://www.apache.org/licenses/LICENSE-2.0 | ||
|
||
Unless required by applicable law or agreed to in writing, software | ||
distributed under the License is distributed on an "AS IS" BASIS, | ||
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | ||
See the License for the specific language governing permissions and | ||
limitations under the License. | ||
#} | ||
{%- extends BASE_HTML -%} | ||
|
||
{% set main_id = 'dev-datagemma' %} | ||
{% set page_id = 'page-dev-datagemma' %} | ||
{% set title = 'Datagemma' %} | ||
{% set is_hide_header_search_bar = 'true' %} | ||
|
||
{% block head %} | ||
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons"> | ||
{% endblock %} | ||
|
||
{% block content %} | ||
<div id="datagemma"></div> | ||
{% endblock %} | ||
|
||
{% block footer %} | ||
<script src={{url_for('static', filename='datagemma.js', t=config['GAE_VERSION'])}}></script> | ||
{% endblock %} | ||
|
Oops, something went wrong.